Interview Cheat Sheet: Full Stack Software Engineer
Everything you need to ace your next interview for your full stack engineering career.
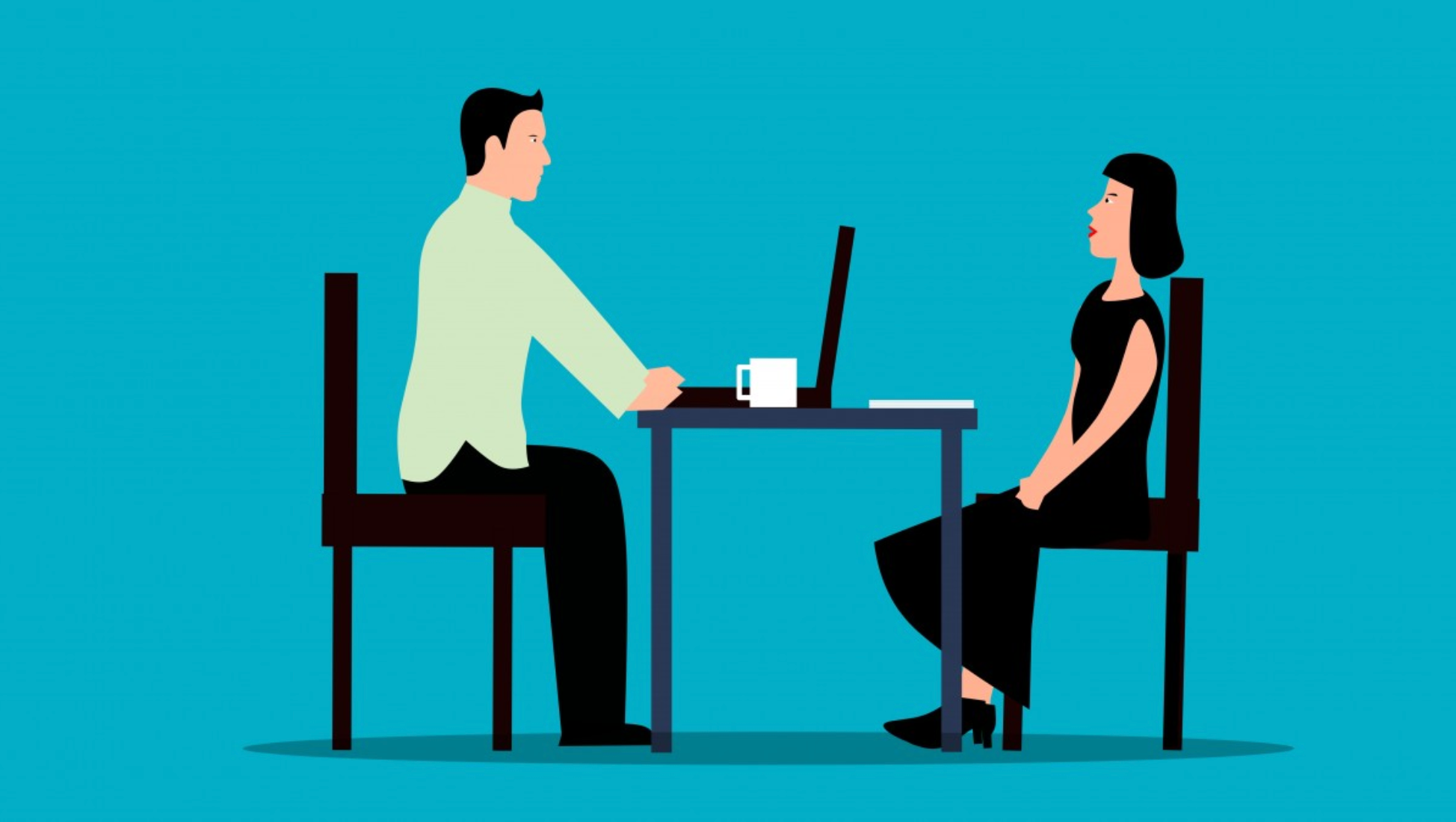
We’ve gone ahead and streamlined your next interview:
Introducing Merit Interview Cheat Sheets. Below, we’ve compiled everything you need to know — from how to prepare, who to talk to, and what to expect — to land your next role as a software engineer.
Keep an eye out for future editions covering other common roles in tech!
- Typical interview process for Full Stack Engineers
- The difference between startup and bit tech interviews
- Technical Assessments - common questions and sample answers
- General interviewing tips
Typical Interview Process for Full Stack Engineers
- Screening Call (30 minutes): The process begins with a screening call conducted by a recruiter or hiring manager. This call assesses your interest in the role, career background, and alignment with the job requirements.
- Technical Assessments (45-60 minutes): Successful candidates move on to technical assessments, which often include coding challenges and system design exercises. These tests evaluate problem-solving skills, coding proficiency, and ability to architect scalable solutions.
- On-Site Interviews (two-to-four 45-minute sessions): Candidates who excel in technical assessments are invited for on-site interviews. These sessions cover technical topics like data structures, algorithms, and potentially specific technologies used by the company. Behavioral interviews assess teamwork and communication skills.
- Final Interview or Offer Discussion (30-40 minutes): Depending on the company, a final interview or additional rounds may occur to finalize the decision. This stage may involve meeting senior leaders and discussing compensation and benefits.
Throughout the process, thorough preparation is essential. Candidates should research the company, practice coding challenges, and demonstrate enthusiasm for the role and cultural fit. This approach maximizes their chances of success as a full stack engineer.
Interviewing at a Startup or for Big Tech
Interviewing as a full stack engineer can vary significantly depending on whether you're targeting a start-up or a big tech company. Each environment offers unique challenges and opportunities that shape the interview process and expectations for candidates.
In a start-up company, the emphasis often lies on versatility and agility. Start-ups typically seek engineers who can wear multiple hats, spanning across various tech stacks and roles; and they care a lot about aptitude combined with a willingness to work independently and iteratively.
Interview processes in start-ups may involve fewer formalities and more emphasis on hands-on skills and problem-solving abilities. Candidates may encounter scenarios where they need to demonstrate not only technical prowess but also creativity and the ability to innovate under limited resources.
Conversely, interviewing with a big tech company tends to be more structured and rigorous. Bigger companies often get significantly more candidates than startups, therefore requiring the actual process and length of the interview to operate as a weeding function. The more difficult it is to follow along, the fewer candidates will make it through.
Big companies also often have established processes, comprehensive technical assessments, and stringent criteria for evaluating candidates. Expectations may include deep expertise in specific technologies, thorough understanding of software engineering principles, and the ability to collaborate within larger teams across diverse projects.
Below, we’ve organized a list of the most important skills you should be able to speak to in your engineering interview process, organized by which environment values them most:
Nailing the Technical Assessment and Onsite Follow-Up
The technical interview for a full stack engineer is designed to evaluate a candidate's depth of technical knowledge, problem-solving abilities, and practical application skills. Typically conducted in multiple rounds, these interviews start with coding assessments where candidates are tasked with solving algorithmic problems that test their understanding of data structures, sorting algorithms, and search algorithms. Candidates may be asked to implement solutions in real-time, either on a whiteboard or using an integrated development environment. System design interviews follow, focusing on candidates' ability to architect scalable and efficient solutions.
Throughout these interviews, candidates are also evaluated on their ability to communicate technical concepts clearly, justify design decisions, and collaborate effectively, reflecting the holistic expectations of a full stack engineer in a tech company environment.
Big Tech
- Data Structures: Mastering data structures is crucial as they form the foundation for efficient algorithm design and problem-solving. Interviewers assess your understanding of these structures to gauge your ability to optimize code performance and handle large datasets.
- Sample Answer: A stack follows Last In First Out (LIFO) principle, whereas a queue follows First In First Out (FIFO) principle. Stacks are used for recursive function calls and backtracking, while queues are suitable for tasks like managing printer queues or handling requests in a web server.
- Likely to happen onsite
- Connect with Merit mentors who are experts on data structures
- Algorithms: Algorithms are essential for solving complex problems efficiently. Mastery of sorting and searching algorithms demonstrates your ability to analyze, design, and implement algorithms that meet performance and scalability requirements.
- Sample Answer: Merge sort divides the array into two halves, recursively sorts each half, and then merges them back together in O(n log n) time. It's stable and efficient for sorting large datasets, making it suitable for applications requiring predictable performance.
- Likely to happen onsite
- Connect with Merit mentors who are experts on algorithms
Startup
- Object-Oriented Programming (OOP): OOP principles are fundamental in start-ups where rapid iteration and code maintainability are critical. Understanding encapsulation, inheritance, and polymorphism allows engineers to build scalable and maintainable codebases.
- Sample Answer: Polymorphism allows objects of different classes to be treated as objects of a common superclass. For example, in a banking application, both SavingsAccount and CheckingAccount classes can inherit from a common Account superclass, allowing methods like ‘calculateinterest()’ to be polymorphic.
- Likely to happen virtually and/or onsite
- Connect with Merit mentors who are experts on OOP
- Systems Design: Start-ups often require engineers to design scalable systems from scratch. Knowledge of system design principles, including load balancing, caching, and database sharding, ensures that the architecture can handle increasing user loads and data volumes. (Systems Design is also commonly part of big tech interviewing!)
- Sample Answer: Utilize a distributed hash table (DHT) for fast lookup and scalability. Store shortened URLs and their original counterparts in a database with efficient indexing for quick retrieval. Implement caching to reduce database load and handle high traffic efficiently.
- Likely to happen virtually
- Connect with Merit mentors who are experts on Systems Design
- Databases: Understanding both SQL and NoSQL databases is crucial in start-ups where data management plays a pivotal role in product development and scalability.
- Sample Answer: SQL databases are ideal for structured data and complex queries requiring ACID transactions, like financial transactions. NoSQL databases, such as MongoDB, are suited for unstructured or semi-structured data and scalable applications needing high availability and partition tolerance, like social media feeds.
- Likely to happen virtually
- Connect with Merit mentors who are experts on Databases
- Web Technologies: Proficiency in web technologies ensures engineers can build robust and user-friendly applications that meet modern web standards and integrate seamlessly with various platforms.
- Sample Answer: JavaScript is a versatile scripting language widely used for web development, allowing for dynamic content and interaction on web pages. TypeScript, on the other hand, is a superset of JavaScript that introduces static typing, allowing developers to catch errors during development rather than at runtime. TypeScript also includes advanced features like interfaces and type annotations, which enhance code maintainability and scalability, making it especially useful for large-scale projects.
- Likely to happen virtually
- Connect with Merit mentors who are experts on Web Technologies
- Testing and Debugging: Thorough testing and debugging skills are essential in start-ups where early-stage products require rapid iteration and quality assurance to meet market demands and user expectations.
- Sample Answer: I write unit tests using frameworks like JUnit to verify individual units of code, ensuring each function behaves as expected. I also consider edge cases and boundary conditions to validate the robustness of the code and prevent regressions during feature enhancements.
- Likely to happen virtually and onsite
- Connect with Merit mentors who are experts on Debugging
Practical Interviewing Advice
- Write clean and easy-to-use code: In technical interviews, demonstrating your ability to write clean, readable, and efficient code is crucial. Clean code not only helps interviewers understand your thought process but also reflects your professionalism and attention to detail. Use meaningful variable names, keep your functions short and focused, and structure your code logically. Commenting is equally important; it provides clarity on complex parts of your code and shows that you can communicate your ideas effectively. Adhering to best practices, such as avoiding hard-coded values and using proper indentation, ensures that your code is maintainable and scalable.
- Write code that is clean and easy for your interviewer to read.
- Comment where necessary and follow best practices.
- Understand the Company and Role:Understanding the company and the role you are applying for is fundamental to tailoring your responses and demonstrating genuine interest, as well as being able to ask good questions to help you understand what kind of projects you’ll be working on. Well-researched questions also allow you to highlight relevant experiences and skills that match their needs. Showing that you have done your homework reflects positively on your dedication and enthusiasm.
- Research the company’s tech stack, products, and culture.
- Understand the specific requirements and responsibilities of the role you are applying for. For instance, ahead of the interview, write out three specific questions about the business or team you’re looking to join.
- Communicate Clearly and Effectively:Clear and effective communication is key during technical interviews. When solving problems, verbalize your thought process and approach. This not only helps the interviewer follow your logic but also demonstrates your problem-solving skills and analytical thinking. Additionally, be ready to discuss your past projects and experiences in detail. Highlight the challenges you faced, how you overcame them, and the impact of your contributions. Being articulate about your experiences shows that you can not only write code but also understand and explain complex systems and decisions.
- Explain your thought process and approach during problem-solving — talk out loud!
- Be prepared to discuss your past projects and experiences in detail.
- Stay Calm and Positive:Technical and onsite interviews can be challenging, and it’s normal to encounter difficult questions. Staying composed helps you think more clearly and reduces the likelihood of making mistakes. If you face a tough problem, take your time to think it through and don’t hesitate to ask clarifying questions — remember, everyone is human! A positive demeanor, even in the face of challenges, leaves a good impression and demonstrates resilience and a growth mindset.
- Keep a positive attitude, even when faced with difficult questions.
- Take your time to think through problems and don’t be afraid to ask clarifying questions.
Successfully navigating a full stack software engineering interview requires a blend of technical expertise, clear communication, and strategic preparation. By understanding the typical interview process, recognizing the differences between start-up and big tech environments, and honing the essential skills outlined in this cheat sheet, candidates can position themselves for success. From mastering data structures and algorithms to demonstrating proficiency in system design and web technologies, preparation is key. Keep these tips in mind, stay composed and confident, and leverage the insights provided to make a lasting impression. Good luck!